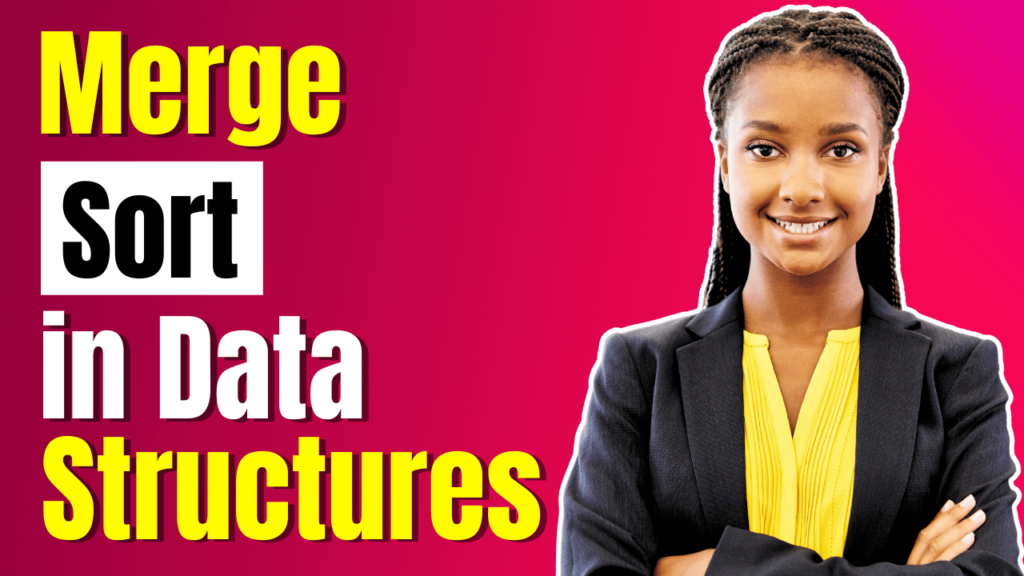
Comparative Analysis: Merge Sort vs. Quick Sort in Algorithm Efficiency
In the world of computer science and algorithm analysis, two sorting algorithms stand out as powerful and widely used techniques: merge sort algorithm and quick sort. These algorithms, while serving the same purpose of arranging elements in a specific order, employ vastly different approaches and exhibit distinct characteristics in terms of efficiency and performance. This article delves into a comprehensive comparative analysis of merge sort and quick sort, highlighting their strengths, weaknesses, and suitability for various scenarios.
Understanding Merge Sort
Merge sort is a divide-and-conquer algorithm that recursively divides the input array into two halves until each subarray contains only one element. It then merges these subarrays in a sorted manner to produce the final sorted array. The key steps involved in the merge sort algorithm are:
-
Divide: Recursively divide the unsorted array into two halves until each subarray contains only one element.
-
Conquer: Sort the individual subarrays by merging them in a sorted manner.
-
Combine: Merge the sorted subarrays back into a single sorted array.
The merging process is the core of the merge sort algorithm, where it compares elements from the two sorted subarrays and places them in the correct order in the final sorted array.
Time Complexity Analysis
The time complexity of merge sort is O(n log n) in the average and worst cases, where n is the number of elements in the input array. This is because the divide step takes logarithmic time, and the conquer step takes linear time for each recursive call.
Space Complexity Analysis
Merge sort has a space complexity of O(n), as it requires an additional temporary array to store the merged subarrays during the sorting process.
Understanding Quick Sort
Quick sort is another divide-and-conquer algorithm that works by partitioning the input array around a pivot element. The algorithm selects a pivot element from the array and rearranges the other elements such that smaller elements are placed before the pivot, and larger elements are placed after the pivot. This process is then repeated recursively on the two partitions until the entire array is sorted.
The key steps involved in the quick sort algorithm are:
-
Partition: Select a pivot element from the array and partition the array into two parts: one with elements smaller than the pivot, and the other with elements larger than the pivot.
-
Sort: Recursively sort the two partitions by repeating the partitioning process.
-
Combine: No explicit combining step is required since the partitioning process itself places the elements in the correct order.
The choice of the pivot element can significantly impact the performance of the quick sort algorithm, as it determines the balance of the partitions.
Time Complexity Analysis
In the average case, when the pivot is chosen optimally (close to the median), the time complexity of quick sort is O(n log n), where n is the number of elements in the input array. However, in the worst-case scenario, when the pivot is always the smallest or largest element, the time complexity degrades to O(n^2).
Space Complexity Analysis
Quick sort has a space complexity of O(log n) in the average case, as it uses recursive function calls to implement the divide-and-conquer approach. However, in the worst-case scenario, when the partitions are highly imbalanced, the space complexity can degrade to O(n) due to the maximum recursion depth.
Comparison of Merge Sort and Quick Sort
Stability
Merge sort is a stable sorting algorithm, meaning it preserves the relative order of equal elements in the input array. Quick sort, on the other hand, is not a stable sorting algorithm by default, as it may rearrange the order of equal elements during the partitioning process.
Space Complexity
Merge sort has a higher space complexity of O(n) due to the additional temporary array required for merging. Quick sort, in contrast, has a lower average space complexity of O(log n) due to its recursive implementation.
Worst-Case Scenarios
While both algorithms have an average time complexity of O(n log n), their worst-case scenarios differ. Merge sort maintains its O(n log n) time complexity even in the worst case, providing consistent performance. Quick sort, however, can degrade to O(n^2) in the worst-case scenario when the partitions are highly imbalanced.
Adaptability to Specific Data Structures
Merge sort can be easily adapted to work with various data structures, such as linked lists or external storage devices like disks, where efficient merging is possible. Quick sort, on the other hand, is typically more suitable for sorting arrays or similar data structures that allow efficient partitioning.
Parallelization
Merge sort lends itself well to parallelization, as the divide and conquer steps can be performed concurrently on different processors or threads. Quick sort, while parallelizable, may require additional synchronization mechanisms to ensure thread safety during the partitioning process.
Use Cases
Merge sort is often preferred when:
-
Stability is a requirement, such as in scenarios where the relative order of equal elements needs to be preserved.
-
Working with linked lists or external storage devices, where efficient merging is possible.
-
Parallelization is desired for improved performance.
Quick sort is often preferred when:
-
Working with arrays or similar data structures that allow efficient partitioning.
-
Average-case performance is prioritized over worst-case scenarios.
-
Space complexity is a concern, and the algorithm needs to operate within limited memory constraints.
Optimizations and Variations
Both merge sort and quick sort have various optimizations and variations that aim to improve their performance or adapt them to specific use cases.
Merge Sort Optimizations
-
Insertion Sort for Small Subarrays: For small subarrays (typically of size 16 or less), using insertion sort instead of merge sort can provide better performance due to reduced overhead.
-
Parallel Merge Sort: By leveraging parallel processing and dividing the work across multiple threads or processors, merge sort can achieve significant performance improvements, especially on large datasets.
Quick Sort Optimizations
-
Pivot Selection Strategies: Choosing an optimal pivot element can greatly improve the performance of quick sort by reducing the imbalance in partitions. Techniques like median-of-three pivot selection or randomized pivot selection can help mitigate worst-case scenarios.
-
Hybrid Approach: Combining quick sort with another sorting algorithm, such as insertion sort for small partitions, can lead to improved performance by reducing the overhead of recursion.
Variations
-
Merge Sort Variations: Examples include natural merge sort, which exploits existing runs (sorted subarrays) in the input data, and in-place merge sort, which reduces the additional space requirement.
-
Quick Sort Variations: Examples include dual-pivot quick sort, which uses two pivots to partition the array, and randomized quick sort, which randomly selects the pivot element to avoid worst-case scenarios.
Applications and Use Cases
Both merge sort and quick sort have found widespread applications in various domains, including:
-
Sorting Algorithms in Programming Languages: These algorithms are commonly implemented in standard libraries of programming languages for sorting arrays, lists, or other data structures.
-
Database Systems: Merge sort and quick sort are used in database management systems for efficient sorting and indexing of data.
-
External Sorting: Merge sort is particularly suitable for sorting large datasets that do not fit in main memory, as it can efficiently merge sorted runs from external storage devices.
-
Parallel and Distributed Computing: The inherent parallelizability of merge sort makes it a popular choice in parallel and distributed computing environments, where tasks can be divided and executed concurrently.
-
Queue data structure Operations: Quick sort is often used in queue implementation for efficient enqueue and dequeue operations, leveraging its ability to partition elements.
Conclusion
In the realm of sorting algorithms, both merge sort and quick sort have proven to be powerful and efficient techniques, each with its own strengths and weaknesses. Merge sort excels in maintaining stability, handling linked lists and external storage devices, and facilitating parallelization, while quick sort shines in its average-case performance, space complexity, and adaptability to array-like data structures.
The choice between these two algorithms depends on various factors, such as the specific requirements of the problem, the characteristics of the input data, and the trade-offs between time and space complexity. In scenarios where stability is crucial, or when working with linked lists or external storage devices, merge sort may be the preferred choice. On the other hand, quick sort might